Table of contents
Open Table of contents
Description
An example project demonstrating user authentication and authorization built with React.js and Go.
It uses Google OAuth 2.0 for secure login and cookies for session management.
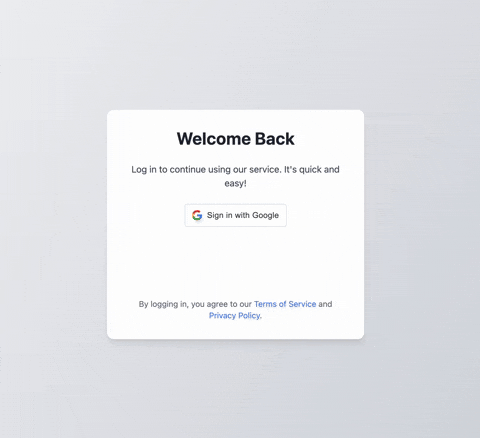
Source code can be found on GitHub.
How OAuth 2.0 Works
OAuth 2.0 is a protocol that allows apps to securely delegate authentication to providers like Google. The flow looks like this:
- The app redirects you to the provider’s login page
- After you log in, the provider asks if you’re okay with sharing specific data (scopes) with the app
- If you agree, it redirects you to the app’s predefined callback URL, passing along the temporary authorization code as a query parameter
- The app exchanges this code for a secure access token and optionally a refresh token, which allows the app to request new access tokens without requiring you to log in again
- Using the access token, the app fetches your data (like your email and name) from the provider’s APIs
Prerequisites
- Install Dependencies:
- Docker
- Node.js and npm for the React frontend.
- Go for the backend.
- Set Up Google OAuth Credentials:
- Create a project in Google Developer Console.
- Generate a Client ID and Client Secret.
- Set the redirect URI to
http://localhost:8100/auth/callback?provider=google
.
Getting Started
-
Clone the Repository:
git clone https://github.com/martishin/react-golang-user-login-oauth cd react-golang-auth
-
Set Up Environment Variables: Copy the .env.example file to .env in the root of the project and update the values as needed:
PORT=8100 DB_HOST=localhost DB_PORT=5432 DB_DATABASE=oauth DB_USERNAME=postgres DB_PASSWORD=postgres GOOGLE_CLIENT_ID=YOUR_GOOGLE_APP_CLIENT_ID GOOGLE_CLIENT_SECRET=YOUR_GOOGLE_CLIENT_SECRET GOOGLE_CALLBACK_URL=http://localhost:8100/auth/callback?provider=google REDIRECT_SECURE=http://localhost:5173/secure SESSION_COOKIE_DOMAIN=localhost ENV=local SESSION_SECRET=YOUR_SESSION_SECRET
-
Start the Database:
docker compose up db -d
-
Start the Backend Server: Navigate to the
server
directory:cd server make run
The backend will be running at http://localhost:8100.
-
Start the Frontend: Navigate to the
client
directory:cd client npm install npm run dev
The frontend will be available at http://localhost:5173.
API Endpoints
Method | Endpoint | Description |
---|---|---|
GET | /auth | Initiate Google OAuth flow |
GET | /auth/callback | Google OAuth callback |
GET | /auth/logout | Log out and clear session |
GET | /api/user | Get authenticated user details |
Tech Stack
- Frontend: React.js, Tailwind CSS
- Backend: Go, Chi, goth
- Database: PostgreSQL
- Auth Provider: Google OAuth 2.0
- Others: Docker